Learn how to develop custom clients for seamless communication with other services.
If you need to integrate your VTEX IO app with external services that aren't covered by VTEX IO's native Clients, creating custom clients can be a powerful solution. Custom clients extend the functionality of VTEX IO Client types, offering benefits like caching and versioning. This guide will walk you through the process of creating custom clients for your VTEX IO apps.
Note that direct communication with APIs is generally discouraged in favor of implementing a dedicated Client. By creating custom Clients, you can extend the capabilities of your VTEX IO applications, opening up opportunities to integrate with a wide range of services seamlessly.
As you develop your custom Client, you will define methods within it. These Client methods are functions responsible for handling the logic needed to execute specific actions. They facilitate interactions with services and APIs, managing requests and responses effectively.
Once you've created your custom Client, you can seamlessly integrate it into your VTEX IO app to execute the implemented methods. While you have the flexibility to incorporate data-handling logic within your Client's methods (e.g., field mapping or data filtering), it's essential to keep the core responsibilities of the Client well-organized.
Before you begin
Before diving into Client development, make sure you have the following prerequisites in place:
- VTEX IO development workspace: Ensure you have a VTEX IO development workspace set up. For more information, refer to Creating a Development workspace.
- TypeScript Familiarity: This guide assumes you have a basic understanding of TypeScript, as we'll be using the
node
Builder to develop with TypeScript. For more information, refer to Typescript's official documentation. - Understanding of Clients: Clients play a crucial role in facilitating interactions between your application and both external and internal services. For more information, refer to Clients.
Instructions
In this guide, we will create an example custom Client for communicating with GitHub APIs. We'll implement the following methods within our Client:
getRepo
: Fetches a GitHub repository given the owner and repository name.getUser
: Retrieves GitHub user data based on a specified GitHub username.createTag
: Creates a new tag on GitHub, considering the repository owner, repository, and tag data.searchRepos
: Searches for GitHub repositories using a specified query.
Step 1 - Setting up your VTEX IO app
-
Start a new VTEX IO app using the
node
builder and open the project using your preferred code editor. -
Install the
@vtex/api
package by running the following command:_10yarn add @vtex/api -
Open the app's
manifest.json
file and add the necessary Policies. Policies are a set of permissions granted to an app, allowing or forbidding it to execute a given set of actions, such as making requests to an external resource. For this example, we will use theoutbound-access
policy to communicate with GitHub APIs (api.github.com
)._10"policies": [_10{_10"name": "outbound-access",_10"attrs": {_10"host": "api.github.com",_10"path": "*"_10}_10},_10]Note that incorrect policies may result in request blocking.
Step 2 - Creating a custom Client
-
Create a folder named
clients
inside thenode
directory. -
Create a TypeScript file for your Client in the
node/clients
directory. Choose a name that easily identifies your Client (e.g.,github.ts
). -
Implement the Typescript class that extends the appropriate Client type from
@vtex/api
. In this example, we will create theGithubClient
. Also, since we are communicating with GitHub's external APIs, we will useExternalClient
. -
Use
InstanceOptions
as needed, configuring options like authentication, timeout, caching, and more based on your requirements._21//node/clients/github.ts_21import {_21ExternalClient,_21IOContext,_21InstanceOptions_21} from "@vtex/api";_21_21export default class GithubClient extends ExternalClient {_21constructor(context: IOContext, options?: InstanceOptions) {_21super("https://api.github.com", context, {_21...options,_21retries: 2,_21headers: {_21Accept: 'application/vnd.github.machine-man-preview+json',_21Authorization: 'Bearer my.cool.jwt'_21}_21});_21}_21_21// We will add the Client-related methods here_21}
Ensure to export the Client from its module (either default or named export).
Step 3 - Implementing Client methods
Within your Client TypeScript file, implement the methods for your Client. You can leverage the methods provided by the HttpClient to build your own methods. For example:
_10//node/clients/github.ts_10public getRepo({owner, repo}: GitRepo) {_10 return this.http.get<ReposGetResponse>(this.routes.repo({owner, repo}),{_10 metric: 'git-repo-get',_10 headers: {_10 Accept: 'application/vnd.github.nebula-preview+json'_10 }_10 })_10}
Refer to the image below, which showcases the complete code of our example:
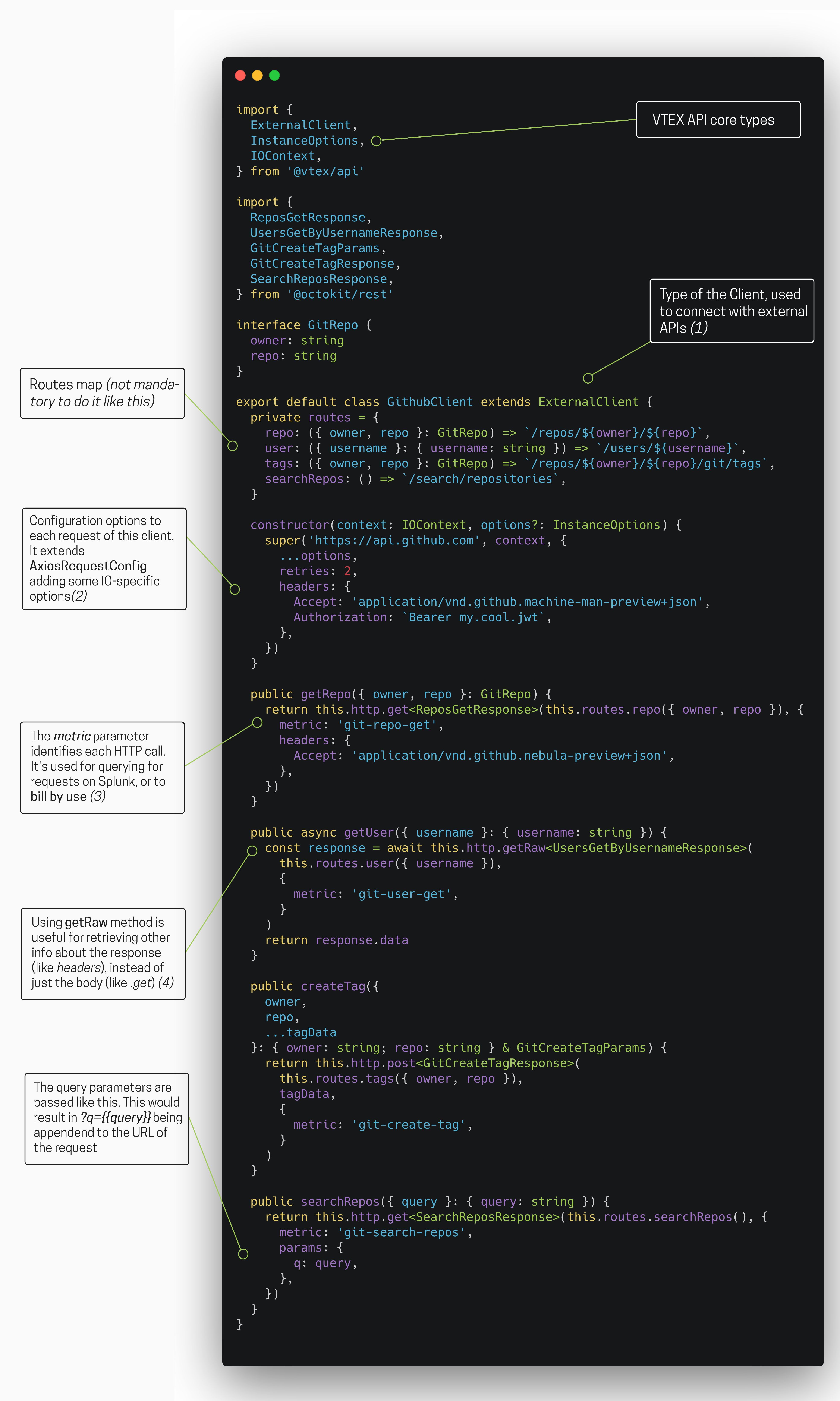
Step 4 - Exporting custom clients
Now that you've created your custom Client, you need to organize and export it for use in your VTEX IO service. Follow these steps:
-
Create an
index.ts
file in thenode/clients
folder. -
Inside the
index.ts
file, import the custom client you created in the previous step. For example:_10import GithubClient from "./github.ts"; -
Define a class called
Clients
that extendsIOClients
. This class is used to organize and configure your custom Clients. Within the Clients class, declare aget
property for each of your custom Clients. This property allows you to access a specific Client by name. In our example, we've created a client namedgithub
that uses theGithubClient
class._10import { IOClients } from "@vtex/api";_10import GithubClient from "./github.ts";_10_10export class Clients extends IOClients {_10public get status() {_10return this.getOrSet("github", GithubClient);_10}_10}
Now that you have developed and exported your custom Client to communicate with the desired service, your Clients can be accessed and used within your VTEX IO service to perform various tasks, such as interacting with external APIs like GitHub. Learn how to use clients effectively in the Using Node Clients guide.
Client types
The @vtex/api
package provides a structured way to create clients.
Type | Use case |
---|---|
AppClient | Communication with other IO Services via HTTP calls. |
AppGraphQLClient | Communication with other IO GraphQL services. |
ExternalClient | Communication with external APIs. |
JanusClient | Communication with VTEX Core Commerce APIs through Janus Router. |
InfraClient | Communication with VTEX IO Infra services. |
Refer to the diagram below to better understand the relationship between Client types.
Note that context
is of type IOContext
and options
is of type InstanceOptions
.
InstanceOptions
The InstanceOptions
table below provides a detailed description of the available options for configuring your HttpClient
instance:
Option | Description |
---|---|
authType | Specifies the authentication type. |
timeout | Sets the request timeout duration in milliseconds. |
memoryCache | Configures a memory cache layer for caching data. |
diskCache | Configures a disk cache layer for caching data. |
baseURL | Defines the base URL for making requests. |
retries | Specifies the number of times a request should be retried in case of failure. |
exponentialTimeoutCoefficient | Configures the coefficient for exponential timeout backoff strategy. |
initialBackoffDelay | Sets the initial delay before starting exponential backoff retries in milliseconds. |
exponentialBackoffCoefficient | Configures the coefficient for exponential backoff retries. |
metrics | Specifies an object for accumulating metrics related to requests. |
concurrency | Defines the maximum number of concurrent requests. |
headers | Sets default headers to be sent with every request. |
params | Sets default query string parameters to be sent with every request. |
middlewares | Configures an array of middleware functions for request processing. |
verbose | Enables or disables verbose logging for requests and responses. |
name | Defines a custom name for the instance. |
serverTimings | Sets server timings for measuring request and response times. |
httpsAgent | Configures the HTTPS agent for making requests over SSL/TLS. |
HttpClient
methods
Below is a table outlining the methods available in the HttpClient
class:
Method | Description |
---|---|
get(url: string, config?: RequestConfig) | Sends an HTTP GET request. |
getRaw(url: string, config?: RequestConfig) | Sends an HTTP GET request. |
getWithBody(url: string, data?: any, config?: RequestConfig) | Sends an HTTP GET request with a request body. |
getBuffer(url: string, config?: RequestConfig) | Sends an HTTP GET request and resolves with the response data as a buffer along with the headers. |
getStream(url: string, config?: RequestConfig) | Sends an HTTP GET request and resolves with the response as a readable stream (of type IncomingMessage ). |
put(url: string, data?: any, config?: RequestConfig) | Sends an HTTP PUT request. |
putRaw(url: string, data?: any, config?: RequestConfig) | Sends an HTTP PUT request. |
post(url: string, data?: any, config?: RequestConfig) | Sends an HTTP POST request. |
postRaw(url: string, data?: any, config?: RequestConfig) | Sends an HTTP POST request. |
patch(url: string, data?: any, config?: RequestConfig) | Sends an HTTP PATCH request. |
head(url: string, config?: RequestConfig) | Sends an HTTP HEAD request and resolves when the request is complete. |
delete(url: string, config?: RequestConfig) | Sends an HTTP DELETE request. |