Now equipped with knowledge on authenticating API calls in VTEX IO, let's dive into the practical aspect of calling VTEX Core Commerce APIs using native Clients. In this guide, we'll specifically leverage the Catalog
client to retrieve SKU details within the VTEX platform.
In VTEX IO, Clients act as abstractions for external services, simplifying external requests in backend services. We strogly recommend using pre-built Clients from the @vtex/clients package for VTEX Core Commerce services and developing custom Clients for external providers (e.g., Google Maps API).
Installing the @vtex/clients
package
The @vtex/clients
package is a TypeScript library featuring pre-configured Clients for accessing VTEX Core Commerce APIs' capabilities. To use it in your app, change to the node
folder and install the @vtex/clients
package by running the command presented in the right panel.
Configuring the Catalog client
Update your app's client node/clients/index.ts
by importing the Catalog
Client from the @vtex/clients
library and exporting it to your Clients
object. From now on, any function in your app can use the Catalog
Client via ctx.clients.catalog
. Also, thanks to TypeScript typing capabilities, autocomplete will become available for the methods exported by the Catalog
client.
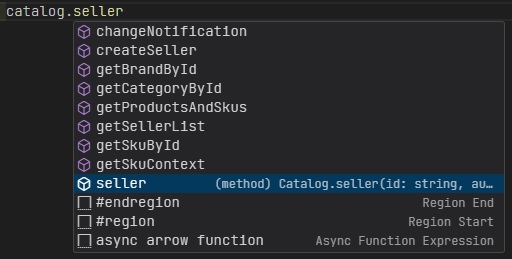
Using the Catalog client
Now, let's use the getSkuById
method from the Catalog
client to retrieve information from a Stock Keeping Unit (SKU) in the VTEX Catalog. To do so, we will update the status
route logic to read the code
parameter as an SKU ID and return SKU details.
Open the node/middlewares/status.ts
file and update it as in the following:
- Extract the
catalog
client from thectx
received in the middleware functions. Learn more about Destructuring on the Mozilla docuentation. - Extract the variable
code
, which will be used as thecode
parameter of our route (/_v/status/:code
). - Call the
getSkuById
method from theCatalog
client. This method will internally call the necessary endpoints of the Catalog API, passing thecode
parameter as the SKU ID to be searched. Also, since this is an asynchronous call, ensure to use theawait
to wait for it.
Declaring the necessary policies
Usually, native Clients are already automatically configured to make authenticated calls using the app token. Nevertheless, you still need to declare that your app will be making requests for some service.
To do so, open the manifest.json
file and add the highlighted code in the right panel to the policies
section. This will allow your app to make calls to the Catalog API.
Fetching the SKU data
With the app linked to your development workspace, copy and send a GET request to the public URL that your service is exposing. Paste in the address bar https://{workspace}--{account}.myvtex.com/_v/status/1
to find information about the SKU ID 1
. The expected result should be a JSON with the SKU data, fetched from the Catalog API.
Installing the @vtex/clients
package
The @vtex/clients
package is a TypeScript library featuring pre-configured Clients for accessing VTEX Core Commerce APIs' capabilities. To use it in your app, change to the node
folder and install the @vtex/clients
package by running the command presented in the right panel.
Configuring the Catalog client
Update your app's client node/clients/index.ts
by importing the Catalog
Client from the @vtex/clients
library and exporting it to your Clients
object. From now on, any function in your app can use the Catalog
Client via ctx.clients.catalog
. Also, thanks to TypeScript typing capabilities, autocomplete will become available for the methods exported by the Catalog
client.
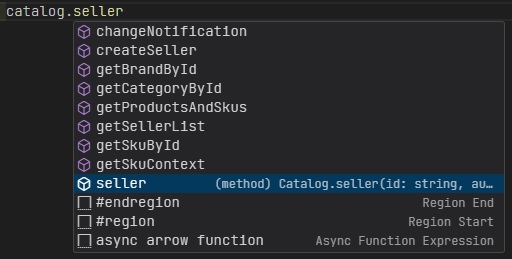
Using the Catalog client
Now, let's use the getSkuById
method from the Catalog
client to retrieve information from a Stock Keeping Unit (SKU) in the VTEX Catalog. To do so, we will update the status
route logic to read the code
parameter as an SKU ID and return SKU details.
Open the node/middlewares/status.ts
file and update it as in the following:
- Extract the
catalog
client from thectx
received in the middleware functions. Learn more about Destructuring on the Mozilla docuentation. - Extract the variable
code
, which will be used as thecode
parameter of our route (/_v/status/:code
). - Call the
getSkuById
method from theCatalog
client. This method will internally call the necessary endpoints of the Catalog API, passing thecode
parameter as the SKU ID to be searched. Also, since this is an asynchronous call, ensure to use theawait
to wait for it.
Declaring the necessary policies
Usually, native Clients are already automatically configured to make authenticated calls using the app token. Nevertheless, you still need to declare that your app will be making requests for some service.
To do so, open the manifest.json
file and add the highlighted code in the right panel to the policies
section. This will allow your app to make calls to the Catalog API.
Fetching the SKU data
With the app linked to your development workspace, copy and send a GET request to the public URL that your service is exposing. Paste in the address bar https://{workspace}--{account}.myvtex.com/_v/status/1
to find information about the SKU ID 1
. The expected result should be a JSON with the SKU data, fetched from the Catalog API.