Overriding a native component
Now that you know how to override a component's prop, learn how to override a native component to use a custom component.
For example, you may want to replace the current
BuyButton
component from the ProductDetails
section to meet your business needs. Currently, when the BuyButton
is clicked, it opens the CartSideBar
as its default behavior: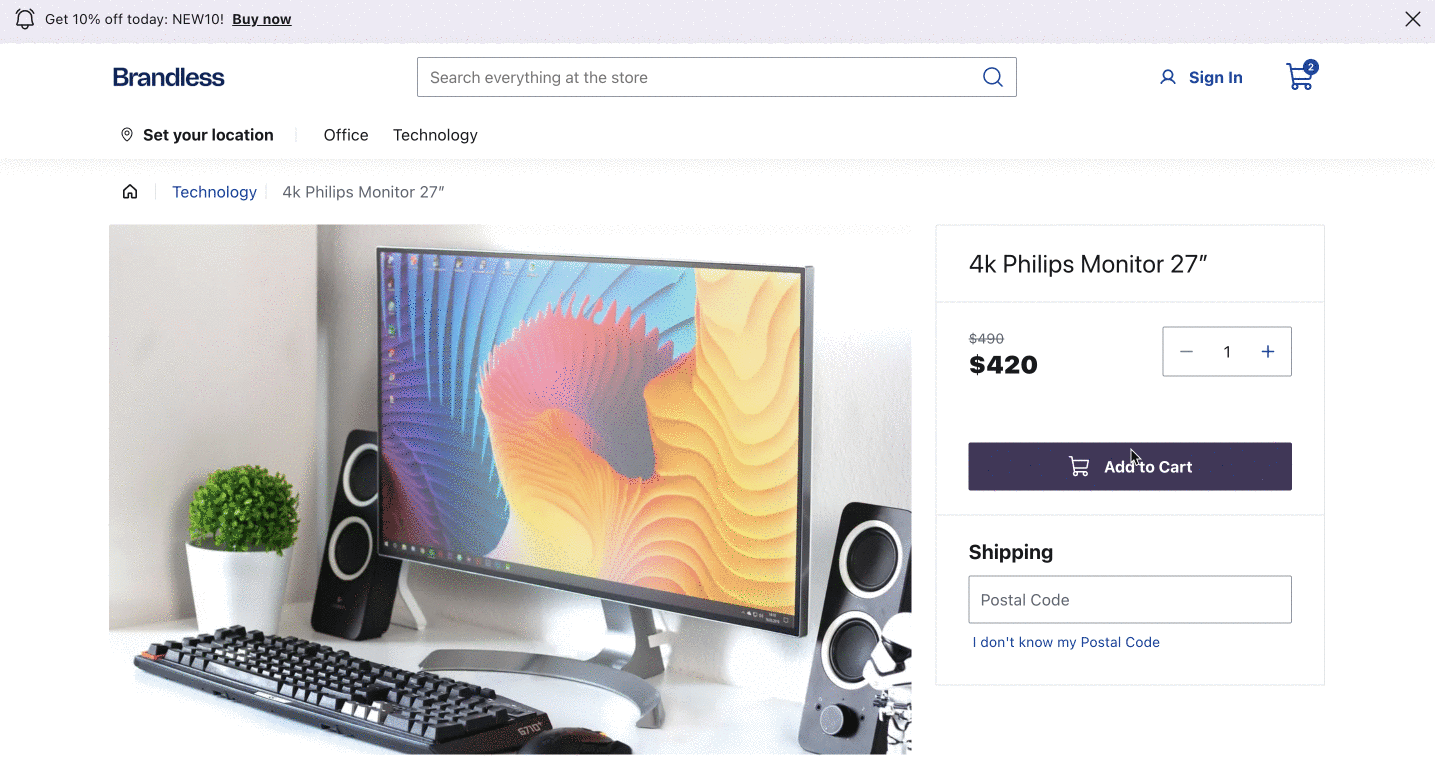
For this guide, we will create a custom BuyButton that displays an
alert
once the user clicks on it.Using overrides does not significantly impact performance. FastStore's handling of props and design tokens is designed for good performance.
Before you begin
Make sure your
@faststore/core
package has the 3.0.0 version or above. If you need to update
it, refer to this release note.Instructions
Step 1 - Setting up the component file
-
After choosing a native section to be customized from the list of available native sections, open your FastStore project in any code editor of your preference.
-
Go to the
src
folder, create thecomponents
folder, and inside it, create thesections
folder. You can run the following command to create them:The naming of thesections
folder is arbitrary, as overrides can be created in any file, since the import is made in thesrc/components/index.tsx
file.macOS and LinuxWindowsbash copy mkdir src/components src/components/sections
Step 2 - Create your custom component
-
Inside the
components
folder, create a file namedCustomBuyButton.tsx
.macOS and LinuxWindows_10touch src/components/CustomBuyButton.tsxWhen creating a custom component, it's important to choose a name that distinguishes it from the native component. For example,Custom{ComponentName}
=CustomBuyButton
. -
Add the following code into
CustomBuyButton.tsx
file:
Step 3 - Setting up the custom section
-
In the
sections
folder, create a new file for your customized section. For example, create a new file namedProductDetailsWithCustomButton.tsx
under thesrc/components/sections
directory. -
Open the
ProductDetailsWithCustomButton.tsx
file and update it with the following code:
Step 4 - Overriding the component
-
Choose a component to override from the list of overridable components of each native section. In this example, we are overriding the
BuyButton
component in theProductDetails
section. -
In the
components
folder, if you don't have already theindex.tsx
file, create the file and add the following: -
Open the terminal, and run
yarn dev
to sync the new custom component to your store preview. -
Open the localhost available after running
yarn dev
. -
Open a PDP and click
New BuyButton
. An alert withHello user
message will appear.
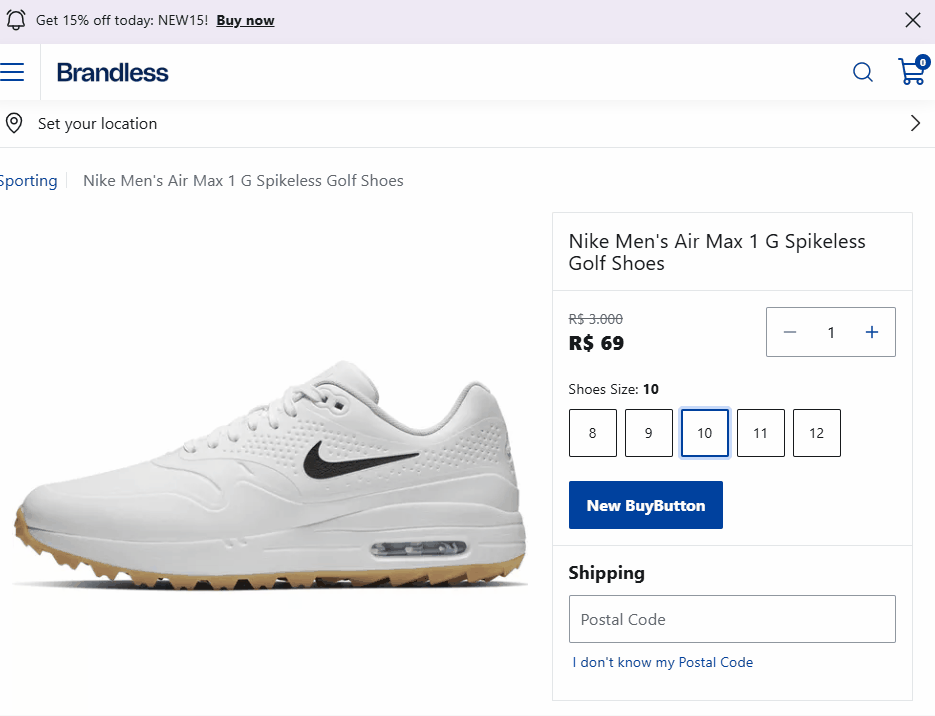
Step 5 - Publishing your changes
If your changes are working locally and you want to publish them in production, follow the steps below:
- Publish your branch with the custom components files.
- Open a pull request in the store's GitHub repository.
- Review the pull request
- Merge the changes from this branch to the
main
one and the new component will be available in the live store.