Cart
The Cart module offers features to manage the store shopping cart. It handles large orders and complex ecommerce operations, such as marketplace integration, coupons, gift options, and promotions.
Cart data storage
Cart data is saved in the browser's IndexedDB, ensuring that users' carts remain saved even when they close the browser. The data is structured as follows:
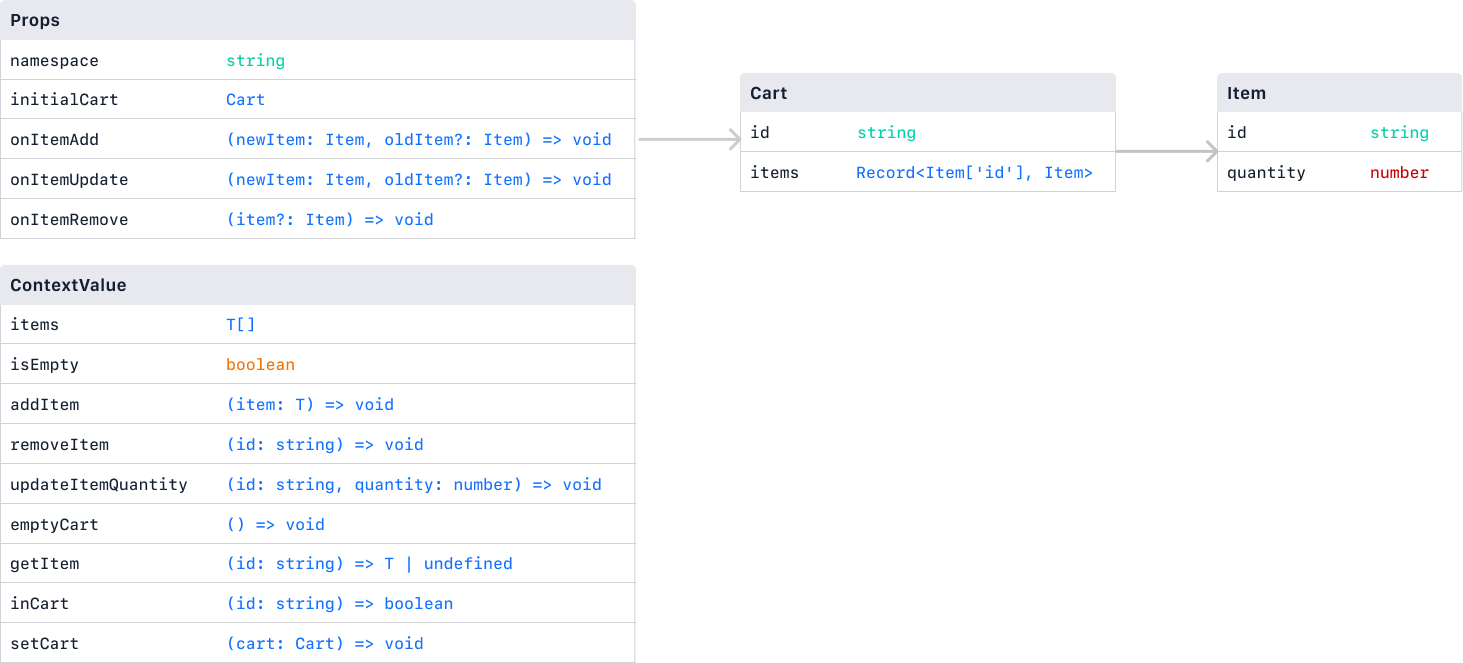
Cart modes
The Cart module provides two modes:
-
Pure (default): Operates on the client side, storing cart data locally and supporting offline use. It lacks server-side validation and may not handle complex cases like unavailable items. Ideal for basic cart functionality.
-
Optimistic: Handles complex ecommerce operations like unavailable items. Validates the cart state on the server using debounced requests.
Pure mode
The Pure mode stores cart data locally in the browser. This allows users to add or remove items and keep their carts even if they close the browser. However, it can't validate items on the server side.
The Pure cart also works offline but doesn't automatically correct errors. For example, if a customer adds an out-of-stock item, the cart won't prevent it. The item will appear in the cart even though it's unavailable.
Implementing Pure mode
To implement the Pure cart mode, follow these steps:
-
Wrap your app with the
CartProvider
component. -
Access the cart using the
useCart
hook:_13import { CartProvider, useCart } from '@faststore/sdk'_13_13// In the App's root component:_13const App = ({ children }) => {_13return <CartProvider>{children}</CartProvider>_13}_13_13// In your component:_13const MyComponent = () => {_13const { items } = useCart()_13_13return <div>Number of items on cart: {items.length}</div>_13}
Optimistic mode
Optimistic mode validates the cart state on the server and handles edge cases like unavailable items. For example, Optimistic mode checks with the store system if a customer tries to add an out-of-stock item. If the product is unavailable, it's removed from the cart, and the customer is notified.
This feature can be implemented in the
optimistic
mode using the validateCart
callback function, which allows developers to make requests and cause side effects to the cart. If the function returns null
, the cart behavior doesn't change. However, if you opt to change the cart state to handle a specific side effect, it must return the new cart state within the callback function.Implementing Optimistic mode
To implement the Optimistic cart mode, follow these steps:
-
Use the
CartProvider
component with theoptimistic
mode. -
Implement the
validateCart
function to handle server-side validation:_27import { CartProvider, useCart, Cart } from '@faststore/sdk'_27_27// In the App's root component:_27const validateCart = async (cart: Cart) => {_27const response = await fetch(...)_27_27if (response) {_27return response_27}_27_27return null_27}_27_27const App = ({children}) => {_27return <CartProvider mode="optimistic" onValidateCart={validateCart}>{children}</CartProvider>_27}_27_27// In your component:_27const MyComponent = () => {_27const { items, isValidating } = useCart()_27_27if (isValidating) {_27return <div>Cart is validating</div>_27}_27_27return <div>Number items in cart: {items.length}</div>_27}